Server Side Google API Access from Android
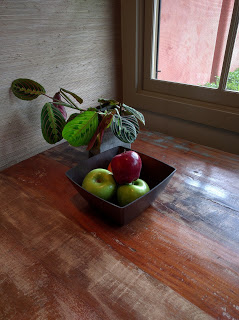
Back in the dark ages I wrote a blog post on using Google Sign In to authorize a server from an Android client, and provided an accompanying gist with an example Activity in it. Recently, someone point out to me that gist was quite out of date, so I updated it to use the latest and greatest Google Sign In APIs on Android, with very pleasant results.
The new version is much easier to understand, and only 2/3 the length of the previous one, without losing any functionality. That’s possible because of some of the features the Identity and Google Play Services teams at Google have added to Google Sign In, and to Google Play Services itself.
Auto manage
Google Play Services is, as the name implies, a separate service on an Android device, and requires a Binder
connection. Back when I wrote my first gist this was wrapped in an API specific client (PlusClient
), and more recently in a generic Google Play Services client (GoogleApiClient
). Managing this connection took quite a lot of code. All of that has been made vastly easier thanks to the auto manage functionality. That effectively hides a very clean implementation of the connection management and error resolution code in a fragment on your activity, and is enabled like this:
Now, the only callback we have to worry about is the OnConnectionFailedListener
. That tells us when there is a more serious problem (for example Google Play Services is missing or being upgraded) - we can’t do much with it other than disable any functionality that needs the service.
The Google Sign In API
Authentication used to be implicit in the GoogleApiClient
connection - it would fail to connect until signed in if using an authenticated API. With the Google Sign In API, this changed - the authentication state and the connection state are separate, easier to manage options.
The net result of this is that checking sign in state is much simpler, and done on that Google Sign In API directly:
Here we also see another newer feature of Google Play Services, the OptionalPendingResult
. PendingResult
is a class which effectively wraps a callback - its kind of like a promise - but OptionalPendingResult
extends that idea to allow for results which may be cached in-process. if opr.isDone
is true you have a result available synchronously, otherwise you can register a callback for an update.
This is particularly convenient here - if the sign in state is cached locally, you can be signed it right away without having to worry about displaying a waiting prompt. If its not, Google Play Services may have to do a network round trip to check whether you have previously signed in to that app, so it probably worth displaying a lightweight spinner or greying out the sign in options until the result comes back.
Integrated auth code retrieval
In the bad old days you had to use GoogleAuthUtil
to retrieve a token, which required a background task and was kind of painful. The Google Sign In API uses a model more similar to the JavaScript implementation, where if you request a token one is delivered with each sign in.
This means that once you get a successful GoogleSignInResult
, you can fetch an authorization code from the GoogleSignInAccount
associated with it.
The gist from before has been updated but for the best introduction check out the complete Google Sign In Sample and documentation.